|
前言
熟悉Windows系统调用可帮我们软件的执行原理
内容
1:熟悉API
API(应用程序编程接口)是微软为开发者提供的一套函数接口,可以用于与系统进行交互、实现一些复杂的功能等。
我们日常在3环写的API如:ReadMemory、CloseHandle、OpenProcess等。其真正的功能实现全部在驱动层,3环中我们使用的只是一个门票,用于通知驱动层我们要实现的功能。而这些API函数从3环的校验代码进入到0环的功能代码的步骤,就称为系统调用。
一些常见的动态链接库如下:
1 Kernel32.dll : 最核心的功能模块,如内存管理,进程线程相关函数等。
2 User32.dll : 用户界面相关的函数。
3 GDI32.dll : 图形设备接口,用于绘制和显示文本等。
4 Ntdll.dll : 大多数API都会通过这个dll进入0环。
2:了解ReadProcess的系统调用过程
接下来我们来了解ReadProcess的调用过程
程序C代码如下
复制代码
1 #include "stdio.h"
2 #include <Windows.h>
3 int main()
4 {
5 int buffer=0;
6 ReadProcessMemory(0,(int*)0x12345678,&buffer,0x4,NULL);
7
8 return 0;
9 }
复制代码
首先查看ReadProcess函数
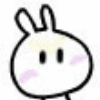
发现调用其ntdll中的NtReadVirtualMemory函数,跟进去
发现将值8A传入eax,然后又接着调用函数,最终通过sysenter指令进入内核
前置知识-内核结构体
TrapFrame
在进入0环时,该结构体用于保存3环寄存器。由windows进行维护。
结构体成员如下:
复制代码
ntdll!_KTRAP_FRAME
+0x000 DbgEbp : Uint4B
+0x004 DbgEip : Uint4B
+0x008 DbgArgMark : Uint4B
+0x00c TempSegCs : Uint2B
+0x00e Logging : UChar
+0x00f FrameType : UChar
+0x010 TempEsp : Uint4B
+0x014 Dr0 : Uint4B
+0x018 Dr1 : Uint4B
+0x01c Dr2 : Uint4B
+0x020 Dr3 : Uint4B
+0x024 Dr6 : Uint4B
+0x028 Dr7 : Uint4B
+0x02c SegGs : Uint4B
+0x030 SegEs : Uint4B
+0x034 SegDs : Uint4B
+0x038 Edx : Uint4B
+0x03c Ecx : Uint4B
+0x040 Eax : Uint4B
+0x044 PreviousPreviousMode : UChar
+0x045 EntropyQueueDpc : UChar
+0x046 NmiMsrIbrs : UChar
+0x046 Reserved1 : UChar
+0x047 PreviousIrql : UChar
+0x048 MxCsr : Uint4B
+0x04c ExceptionList : Ptr32 _EXCEPTION_REGISTRATION_RECORD
+0x050 SegFs : Uint4B
+0x054 Edi : Uint4B
+0x058 Esi : Uint4B
+0x05c Ebx : Uint4B
+0x060 Ebp : Uint4B
+0x064 ErrCode : Uint4B
+0x068 Eip : Uint4B
+0x06c SegCs : Uint4B
+0x070 EFlags : Uint4B
+0x074 HardwareEsp : Uint4B
+0x078 HardwareSegSs : Uint4B
+0x07c V86Es : Uint4B
+0x080 V86Ds : Uint4B
+0x084 V86Fs : Uint4B
+0x088 V86Gs : Uint4B
复制代码
KPCR
在3环时,FS指向TEB结构。在0环时,FS指向KPCR结构。该结构体用于描述对应的CPU的状态。(一核对应一个KPCR)
windbg中输入 dd KeNumberProcessor 查看核数
windbg中输入 dd KiProcessorBlock LXXX 查看每个核的KPCR结构地址。
结构体成员:
复制代码
ntdll!_KPCR
+0x000 NtTib : _NT_TIB
+0x000 Used_ExceptionList : Ptr32 _EXCEPTION_REGISTRATION_RECORD
+0x004 Used_StackBase : Ptr32 Void
+0x008 MxCsr : Uint4B
+0x00c TssCopy : Ptr32 Void
+0x010 ContextSwitches : Uint4B
+0x014 SetMemberCopy : Uint4B
+0x018 Used_Self : Ptr32 Void
+0x01c SelfPcr : Ptr32 _KPCR
+0x020 Prcb : Ptr32 _KPRCB
+0x024 Irql : UChar
+0x028 IRR : Uint4B
+0x02c IrrActive : Uint4B
+0x030 IDR : Uint4B
+0x034 KdVersionBlock : Ptr32 Void
+0x038 IDT : Ptr32 _KIDTENTRY
+0x03c GDT : Ptr32 _KGDTENTRY
+0x040 TSS : Ptr32 _KTSS
+0x044 MajorVersion : Uint2B
+0x046 MinorVersion : Uint2B
+0x048 SetMember : Uint4B
+0x04c StallScaleFactor : Uint4B
+0x050 SpareUnused : UChar
+0x051 Number : UChar
+0x052 Spare0 : UChar
+0x053 SecondLevelCacheAssociativity : UChar
+0x054 VdmAlert : Uint4B
+0x058 KernelReserved : [14] Uint4B
+0x090 SecondLevelCacheSize : Uint4B
+0x094 HalReserved : [16] Uint4B
+0x0d4 InterruptMode : Uint4B
+0x0d8 Spare1 : UChar
+0x0dc KernelReserved2 : [17] Uint4B
+0x120 PrcbData : _KPRCB
复制代码
KPRCB
结构体成员:
复制代码
ntdll!_KPRCB
+0x000 MinorVersion : Uint2B
+0x002 MajorVersion : Uint2B
+0x004 CurrentThread : Ptr32 _KTHREAD
+0x008 NextThread : Ptr32 _KTHREAD
+0x00c IdleThread : Ptr32 _KTHREAD
+0x010 LegacyNumber : UChar
+0x011 NestingLevel : UChar
+0x012 BuildType : Uint2B
+0x014 CpuType : Char
+0x015 CpuID : Char
+0x016 CpuStep : Uint2B
+0x016 CpuStepping : UChar
+0x017 CpuModel : UChar
+0x018 ProcessorState : _KPROCESSOR_STATE
+0x338 ParentNode : Ptr32 _KNODE
+0x33c PriorityState : Ptr32 Char
+0x340 KernelReserved : [14] Uint4B
+0x378 HalReserved : [16] Uint4B
+0x3b8 CFlushSize : Uint4B
+0x3bc CoresPerPhysicalProcessor : UChar
+0x3bd LogicalProcessorsPerCore : UChar
+0x3be CpuVendor : UChar
+0x3bf PrcbPad0 : [1] UChar
+0x3c0 MHz : Uint4B
+0x3c4 GroupIndex : UChar
+0x3c5 Group : UChar
+0x3c6 PrcbPad05 : [2] UChar
+0x3c8 GroupSetMember : Uint4B
+0x3cc Number : Uint4B
+0x3d0 ClockOwner : UChar
+0x3d1 PendingTickFlags : UChar
+0x3d1 PendingTick : Pos 0, 1 Bit
+0x3d1 PendingBackupTick : Pos 1, 1 Bit
+0x3d2 PrcbPad10 : [70] UChar
+0x418 LockQueue : [17] _KSPIN_LOCK_QUEUE
+0x4a0 InterruptCount : Uint4B
+0x4a4 KernelTime : Uint4B
+0x4a8 UserTime : Uint4B
+0x4ac DpcTime : Uint4B
+0x4b0 DpcTimeCount : Uint4B
+0x4b4 InterruptTime : Uint4B
+0x4b8 AdjustDpcThreshold : Uint4B
+0x4bc PageColor : Uint4B
+0x4c0 DebuggerSavedIRQL : UChar
+0x4c1 NodeColor : UChar
+0x4c2 DeepSleep : UChar
+0x4c3 TbFlushListActive : UChar
+0x4c4 CachedStack : Ptr32 Void
+0x4c8 NodeShiftedColor : Uint4B
+0x4cc SecondaryColorMask : Uint4B
+0x4d0 DpcTimeLimit : Uint4B
+0x4d4 MmInternal : Ptr32 Void
+0x4d8 PrcbFlags : _KPRCBFLAG
+0x4dc SchedulerAssist : Ptr32 Void
+0x4e0 CcFastReadNoWait : Uint4B
+0x4e4 CcFastReadWait : Uint4B
+0x4e8 CcFastReadNotPossible : Uint4B
+0x4ec CcCopyReadNoWait : Uint4B
+0x4f0 CcCopyReadWait : Uint4B
+0x4f4 CcCopyReadNoWaitMiss : Uint4B
+0x4f8 MmSpinLockOrdering : Int4B
+0x4fc IoReadOperationCount : Int4B
+0x500 IoWriteOperationCount : Int4B
+0x504 IoOtherOperationCount : Int4B
+0x508 IoReadTransferCount : _LARGE_INTEGER
+0x510 IoWriteTransferCount : _LARGE_INTEGER
+0x518 IoOtherTransferCount : _LARGE_INTEGER
+0x520 CcFastMdlReadNoWait : Uint4B
+0x524 CcFastMdlReadWait : Uint4B
+0x528 CcFastMdlReadNotPossible : Uint4B
+0x52c CcMapDataNoWait : Uint4B
+0x530 CcMapDataWait : Uint4B
+0x534 CcPinMappedDataCount : Uint4B
+0x538 CcPinReadNoWait : Uint4B
+0x53c CcPinReadWait : Uint4B
+0x540 CcMdlReadNoWait : Uint4B
+0x544 CcMdlReadWait : Uint4B
+0x548 CcLazyWriteHotSpots : Uint4B
+0x54c CcLazyWriteIos : Uint4B
+0x550 CcLazyWritePages : Uint4B
+0x554 CcDataFlushes : Uint4B
+0x558 CcDataPages : Uint4B
+0x55c CcLostDelayedWrites : Uint4B
+0x560 CcFastReadResourceMiss : Uint4B
+0x564 CcCopyReadWaitMiss : Uint4B
+0x568 CcFastMdlReadResourceMiss : Uint4B
+0x56c CcMapDataNoWaitMiss : Uint4B
+0x570 CcMapDataWaitMiss : Uint4B
+0x574 CcPinReadNoWaitMiss : Uint4B
+0x578 CcPinReadWaitMiss : Uint4B
+0x57c CcMdlReadNoWaitMiss : Uint4B
+0x580 CcMdlReadWaitMiss : Uint4B
+0x584 CcReadAheadIos : Uint4B
+0x588 KeAlignmentFixupCount : Uint4B
+0x58c KeExceptionDispatchCount : Uint4B
+0x590 KeSystemCalls : Uint4B
+0x594 AvailableTime : Uint4B
+0x598 PrcbPad22 : [2] Uint4B
+0x5a0 PPLookasideList : [16] _PP_LOOKASIDE_LIST
+0x620 PPNxPagedLookasideList : [32] _GENERAL_LOOKASIDE_POOL
+0xf20 PPNPagedLookasideList : [32] _GENERAL_LOOKASIDE_POOL
+0x1820 PPPagedLookasideList : [32] _GENERAL_LOOKASIDE_POOL
+0x2120 PacketBarrier : Int4B
+0x2124 ReverseStall : Int4B
+0x2128 IpiFrame : Ptr32 Void
+0x212c PrcbPad3 : [52] UChar
+0x2160 CurrentPacket : [3] Ptr32 Void
+0x216c TargetSet : Uint4B
+0x2170 WorkerRoutine : Ptr32 void
+0x2174 IpiFrozen : Uint4B
+0x2178 PrcbPad4 : [40] UChar
+0x21a0 RequestSummary : Uint4B
+0x21a4 TargetCount : Int4B
+0x21a8 PrcbPad94 : [1] Uint8B
+0x21b0 TrappedSecurityDomain : Uint8B
+0x21b8 BpbState : UChar
+0x21b8 BpbCpuIdle : Pos 0, 1 Bit
+0x21b8 BpbFlushRsbOnTrap : Pos 1, 1 Bit
+0x21b8 BpbIbpbOnReturn : Pos 2, 1 Bit
+0x21b8 BpbIbpbOnTrap : Pos 3, 1 Bit
+0x21b8 BpbReserved : Pos 4, 4 Bits
+0x21b9 BpbFeatures : UChar
+0x21b9 BpbClearOnIdle : Pos 0, 1 Bit
+0x21b9 BpbEnabled : Pos 1, 1 Bit
+0x21b9 BpbSmep : Pos 2, 1 Bit
+0x21b9 BpbFeaturesReserved : Pos 3, 5 Bits
+0x21ba BpbCurrentSpecCtrl : UChar
+0x21bb BpbKernelSpecCtrl : UChar
+0x21bc BpbNmiSpecCtrl : UChar
+0x21bd BpbUserSpecCtrl : UChar
+0x21be PrcbPad49 : [2] UChar
+0x21c0 ProcessorSignature : Uint4B
+0x21c4 ProcessorFlags : Uint4B
+0x21c8 PrcbPad50 : [8] UChar
+0x21d0 InterruptLastCount : Uint4B
+0x21d4 InterruptRate : Uint4B
+0x21d8 DeviceInterrupts : Uint4B
+0x21dc IsrDpcStats : Ptr32 Void
+0x21e0 DpcData : [2] _KDPC_DATA
+0x2210 DpcStack : Ptr32 Void
+0x2214 MaximumDpcQueueDepth : Int4B
+0x2218 DpcRequestRate : Uint4B
+0x221c MinimumDpcRate : Uint4B
+0x2220 DpcLastCount : Uint4B
+0x2224 PrcbLock : Uint4B
+0x2228 DpcGate : _KGATE
+0x2238 IdleState : UChar
+0x2239 QuantumEnd : UChar
+0x223a DpcRoutineActive : UChar
+0x223b IdleSchedule : UChar
+0x223c DpcRequestSummary : Int4B
+0x223c DpcRequestSlot : [2] Int2B
+0x223c NormalDpcState : Int2B
+0x223e ThreadDpcState : Int2B
+0x223c DpcNormalProcessingActive : Pos 0, 1 Bit
+0x223c DpcNormalProcessingRequested : Pos 1, 1 Bit
+0x223c DpcNormalThreadSignal : Pos 2, 1 Bit
+0x223c DpcNormalTimerExpiration : Pos 3, 1 Bit
+0x223c DpcNormalDpcPresent : Pos 4, 1 Bit
+0x223c DpcNormalLocalInterrupt : Pos 5, 1 Bit
+0x223c DpcNormalSpare : Pos 6, 10 Bits
+0x223c DpcThreadActive : Pos 16, 1 Bit
+0x223c DpcThreadRequested : Pos 17, 1 Bit
+0x223c DpcThreadSpare : Pos 18, 14 Bits
+0x2240 LastTick : Uint4B
+0x2244 PeriodicCount : Uint4B
+0x2248 PeriodicBias : Uint4B
+0x224c ClockInterrupts : Uint4B
+0x2250 ReadyScanTick : Uint4B
+0x2254 GroupSchedulingOverQuota : UChar
+0x2255 ThreadDpcEnable : UChar
+0x2256 PrcbPad41 : [6] UChar
+0x2260 TimerTable : _KTIMER_TABLE
+0x3ab0 PrcbPad92 : [12] Uint4B
+0x3ae0 CallDpc : _KDPC
+0x3b00 ClockKeepAlive : Int4B
+0x3b04 PrcbPad6 : [4] UChar
+0x3b08 DpcWatchdogPeriod : Int4B
+0x3b0c DpcWatchdogCount : Int4B
+0x3b10 KeSpinLockOrdering : Int4B
+0x3b14 DpcWatchdogProfileCumulativeDpcThreshold : Uint4B
+0x3b18 QueueIndex : Uint4B
+0x3b1c DeferredReadyListHead : _SINGLE_LIST_ENTRY
+0x3b20 ReadySummary : Uint4B
+0x3b24 AffinitizedSelectionMask : Int4B
+0x3b28 WaitLock : Uint4B
+0x3b2c WaitListHead : _LIST_ENTRY
+0x3b34 ScbOffset : Uint4B
+0x3b38 ReadyThreadCount : Uint4B
+0x3b40 StartCycles : Uint8B
+0x3b48 TaggedCyclesStart : Uint8B
+0x3b50 TaggedCycles : [3] Uint8B
+0x3b68 CycleTime : Uint8B
+0x3b70 AffinitizedCycles : Uint8B
+0x3b78 ImportantCycles : Uint8B
+0x3b80 UnimportantCycles : Uint8B
+0x3b88 ReadyQueueExpectedRunTime : Uint8B
+0x3b90 HighCycleTime : Uint4B
+0x3b98 Cycles : [4] [2] Uint8B
+0x3bd8 PrcbPad71 : Uint4B
+0x3bdc DpcWatchdogSequenceNumber : Uint4B
+0x3be0 DispatcherReadyListHead : [32] _LIST_ENTRY
+0x3ce0 ChainedInterruptList : Ptr32 Void
+0x3ce4 LookasideIrpFloat : Int4B
+0x3ce8 ScbQueue : _RTL_RB_TREE
+0x3cf0 ScbList : _LIST_ENTRY
+0x3cf8 MmPageFaultCount : Int4B
+0x3cfc MmCopyOnWriteCount : Int4B
+0x3d00 MmTransitionCount : Int4B
+0x3d04 MmCacheTransitionCount : Int4B
+0x3d08 MmDemandZeroCount : Int4B
+0x3d0c MmPageReadCount : Int4B
+0x3d10 MmPageReadIoCount : Int4B
+0x3d14 MmCacheReadCount : Int4B
+0x3d18 MmCacheIoCount : Int4B
+0x3d1c MmDirtyPagesWriteCount : Int4B
+0x3d20 MmDirtyWriteIoCount : Int4B
+0x3d24 MmMappedPagesWriteCount : Int4B
+0x3d28 MmMappedWriteIoCount : Int4B
+0x3d2c CachedCommit : Uint4B
+0x3d30 CachedResidentAvailable : Uint4B
+0x3d34 HyperPte : Ptr32 Void
+0x3d38 PrcbPad8 : [4] UChar
+0x3d3c VendorString : [13] UChar
+0x3d49 InitialApicId : UChar
+0x3d4a LogicalProcessorsPerPhysicalProcessor : UChar
+0x3d4b PrcbPad9 : [1] UChar
+0x3d50 FeatureBits : Uint8B
+0x3d58 UpdateSignature : _LARGE_INTEGER
+0x3d60 IsrTime : Uint8B
+0x3d68 GenerationTarget : Uint8B
+0x3d70 PowerState : _PROCESSOR_POWER_STATE
+0x3f18 ForceIdleDpc : _KDPC
+0x3f38 PrcbPad91 : [8] Uint4B
+0x3f58 DpcRuntimeHistoryHashTable : Ptr32 _RTL_HASH_TABLE
+0x3f5c DpcRuntimeHistoryHashTableCleanupDpc : Ptr32 _KDPC
+0x3f60 CurrentDpcRuntimeHistoryCached : Uint8B
+0x3f68 CurrentDpcStartTime : Uint8B
+0x3f70 CurrentDpcRoutine : Ptr32 void
+0x3f74 DpcWatchdogProfileSingleDpcThreshold : Uint4B
+0x3f78 DpcWatchdogDpc : _KDPC
+0x3f98 DpcWatchdogTimer : _KTIMER
+0x3fc0 HypercallPageList : _SLIST_HEADER
+0x3fc8 HypercallCachedPages : Ptr32 Void
+0x3fcc VirtualApicAssist : Ptr32 Void
+0x3fd0 StatisticsPage : Ptr32 Uint8B
+0x3fd4 Cache : [5] _CACHE_DESCRIPTOR
+0x4010 CacheCount : Uint4B
+0x4014 PackageProcessorSet : _KAFFINITY_EX
+0x4020 SharedReadyQueueMask : Uint4B
+0x4024 SharedReadyQueue : Ptr32 _KSHARED_READY_QUEUE
+0x4028 SharedQueueScanOwner : Uint4B
+0x402c CoreProcessorSet : Uint4B
+0x4030 ScanSiblingMask : Uint4B
+0x4034 LLCMask : Uint4B
+0x4038 CacheProcessorMask : [5] Uint4B
+0x404c ScanSiblingIndex : Uint4B
+0x4050 WheaInfo : Ptr32 Void
+0x4054 EtwSupport : Ptr32 Void
+0x4058 InterruptObjectPool : _SLIST_HEADER
+0x4060 DpcWatchdogProfile : Ptr32 Ptr32 Void
+0x4064 DpcWatchdogProfileCurrentEmptyCapture : Ptr32 Ptr32 Void
+0x4068 PackageId : Uint4B
+0x406c PteBitCache : Uint4B
+0x4070 PteBitOffset : Uint4B
+0x4074 PrcbPad93 : Uint4B
+0x4078 ProcessorProfileControlArea : Ptr32 _PROCESSOR_PROFILE_CONTROL_AREA
+0x407c ProfileEventIndexAddress : Ptr32 Void
+0x4080 TimerExpirationDpc : _KDPC
+0x40a0 SynchCounters : _SYNCH_COUNTERS
+0x4158 FsCounters : _FILESYSTEM_DISK_COUNTERS
+0x4168 Context : Ptr32 _CONTEXT
+0x416c ContextFlagsInit : Uint4B
+0x4170 ExtendedState : Ptr32 _XSAVE_AREA
+0x4174 EntropyTimingState : _KENTROPY_TIMING_STATE
+0x429c IsrStack : Ptr32 Void
+0x42a0 VectorToInterruptObject : [208] Ptr32 _KINTERRUPT
+0x45e0 AbSelfIoBoostsList : _SINGLE_LIST_ENTRY
+0x45e4 AbPropagateBoostsList : _SINGLE_LIST_ENTRY
+0x45e8 AbDpc : _KDPC
+0x4608 IoIrpStackProfilerCurrent : _IOP_IRP_STACK_PROFILER
+0x465c IoIrpStackProfilerPrevious : _IOP_IRP_STACK_PROFILER
+0x46b0 TimerExpirationTrace : [16] _KTIMER_EXPIRATION_TRACE
+0x47b0 TimerExpirationTraceCount : Uint4B
+0x47b4 ExSaPageArray : Ptr32 Void
+0x47b8 ExtendedSupervisorState : Ptr32 _XSAVE_AREA_HEADER
+0x47bc PrcbPad100 : [9] Uint4B
+0x47e0 LocalSharedReadyQueue : _KSHARED_READY_QUEUE
+0x4920 Mailbox : Ptr32 _REQUEST_MAILBOX
+0x4924 PrcbPad : [1468] UChar
+0x4ee0 KernelDirectoryTableBase : Uint4B
+0x4ee4 EspBaseShadow : Uint4B
+0x4ee8 UserEspShadow : Uint4B
+0x4eec ShadowFlags : Uint4B
+0x4ef0 UserDS : Uint4B
+0x4ef4 UserES : Uint4B
+0x4ef8 UserFS : Uint4B
+0x4efc EspIretd : Ptr32 Void
+0x4f00 RestoreSegOption : Uint4B
+0x4f04 SavedEsi : Uint4B
+0x4f08 PrcbShadowPad : Uint4B
+0x4f0c TaskSwitchCount : Uint4B
+0x4f10 DbgLogs : [512] Uint4B
+0x5710 DbgCount : Uint4B
+0x5714 PrcbPadRemainingPage : [499] Uint4B
+0x5ee0 RequestMailbox : [1] _REQUEST_MAILBOX
复制代码
ETHREAD
线程结构体,每一个线程都有一个线程结构体,用于描述对应线程的各种属性。
结构体成员
复制代码
1 ntdll!_ETHREAD
2 +0x000 Tcb : _KTHREAD
3 +0x280 CreateTime : _LARGE_INTEGER
4 +0x288 ExitTime : _LARGE_INTEGER
5 +0x288 KeyedWaitChain : _LIST_ENTRY
6 +0x290 ChargeOnlySession : Ptr32 Void
7 +0x294 PostBlockList : _LIST_ENTRY
8 +0x294 ForwardLinkShadow : Ptr32 Void
9 +0x298 StartAddress : Ptr32 Void
10 +0x29c TerminationPort : Ptr32 _TERMINATION_PORT
11 +0x29c ReaperLink : Ptr32 _ETHREAD
12 +0x29c KeyedWaitValue : Ptr32 Void
13 +0x2a0 ActiveTimerListLock : Uint4B
14 +0x2a4 ActiveTimerListHead : _LIST_ENTRY
15 +0x2ac Cid : _CLIENT_ID
16 +0x2b4 KeyedWaitSemaphore : _KSEMAPHORE
17 +0x2b4 AlpcWaitSemaphore : _KSEMAPHORE
18 +0x2c8 ClientSecurity : _PS_CLIENT_SECURITY_CONTEXT
19 +0x2cc IrpList : _LIST_ENTRY
20 +0x2d4 TopLevelIrp : Uint4B
21 +0x2d8 DeviceToVerify : Ptr32 _DEVICE_OBJECT
22 +0x2dc Win32StartAddress : Ptr32 Void
23 +0x2e0 LegacyPowerObject : Ptr32 Void
24 +0x2e4 ThreadListEntry : _LIST_ENTRY
25 +0x2ec RundownProtect : _EX_RUNDOWN_REF
26 +0x2f0 ThreadLock : _EX_PUSH_LOCK
27 +0x2f4 ReadClusterSize : Uint4B
28 +0x2f8 MmLockOrdering : Int4B
29 +0x2fc CrossThreadFlags : Uint4B
30 +0x2fc Terminated : Pos 0, 1 Bit
31 +0x2fc ThreadInserted : Pos 1, 1 Bit
32 +0x2fc HideFromDebugger : Pos 2, 1 Bit
33 +0x2fc ActiveImpersonationInfo : Pos 3, 1 Bit
34 +0x2fc HardErrorsAreDisabled : Pos 4, 1 Bit
35 +0x2fc BreakOnTermination : Pos 5, 1 Bit
36 +0x2fc SkipCreationMsg : Pos 6, 1 Bit
37 +0x2fc SkipTerminationMsg : Pos 7, 1 Bit
38 +0x2fc CopyTokenOnOpen : Pos 8, 1 Bit
39 +0x2fc ThreadIoPriority : Pos 9, 3 Bits
40 +0x2fc ThreadPagePriority : Pos 12, 3 Bits
41 +0x2fc RundownFail : Pos 15, 1 Bit
42 +0x2fc UmsForceQueueTermination : Pos 16, 1 Bit
43 +0x2fc IndirectCpuSets : Pos 17, 1 Bit
44 +0x2fc DisableDynamicCodeOptOut : Pos 18, 1 Bit
45 +0x2fc ExplicitCaseSensitivity : Pos 19, 1 Bit
46 +0x2fc PicoNotifyExit : Pos 20, 1 Bit
47 +0x2fc DbgWerUserReportActive : Pos 21, 1 Bit
48 +0x2fc ForcedSelfTrimActive : Pos 22, 1 Bit
49 +0x2fc SamplingCoverage : Pos 23, 1 Bit
50 +0x2fc ReservedCrossThreadFlags : Pos 24, 8 Bits
51 +0x300 SameThreadPassiveFlags : Uint4B
52 +0x300 ActiveExWorker : Pos 0, 1 Bit
53 +0x300 MemoryMaker : Pos 1, 1 Bit
54 +0x300 StoreLockThread : Pos 2, 2 Bits
55 +0x300 ClonedThread : Pos 4, 1 Bit
56 +0x300 KeyedEventInUse : Pos 5, 1 Bit
57 +0x300 SelfTerminate : Pos 6, 1 Bit
58 +0x300 RespectIoPriority : Pos 7, 1 Bit
59 +0x300 ActivePageLists : Pos 8, 1 Bit
60 +0x300 SecureContext : Pos 9, 1 Bit
61 +0x300 ZeroPageThread : Pos 10, 1 Bit
62 +0x300 WorkloadClass : Pos 11, 1 Bit
63 +0x300 ReservedSameThreadPassiveFlags : Pos 12, 20 Bits
64 +0x304 SameThreadApcFlags : Uint4B
65 +0x304 OwnsProcessAddressSpaceExclusive : Pos 0, 1 Bit
66 +0x304 OwnsProcessAddressSpaceShared : Pos 1, 1 Bit
67 +0x304 HardFaultBehavior : Pos 2, 1 Bit
68 +0x304 StartAddressInvalid : Pos 3, 1 Bit
69 +0x304 EtwCalloutActive : Pos 4, 1 Bit
70 +0x304 SuppressSymbolLoad : Pos 5, 1 Bit
71 +0x304 Prefetching : Pos 6, 1 Bit
72 +0x304 OwnsVadExclusive : Pos 7, 1 Bit
73 +0x305 SystemPagePriorityActive : Pos 0, 1 Bit
74 +0x305 SystemPagePriority : Pos 1, 3 Bits
75 +0x305 AllowUserWritesToExecutableMemory : Pos 4, 1 Bit
76 +0x305 AllowKernelWritesToExecutableMemory : Pos 5, 1 Bit
77 +0x305 OwnsVadShared : Pos 6, 1 Bit
78 +0x308 CacheManagerActive : UChar
79 +0x309 DisablePageFaultClustering : UChar
80 +0x30a ActiveFaultCount : UChar
81 +0x30b LockOrderState : UChar
82 +0x30c PerformanceCountLowReserved : Uint4B
83 +0x310 PerformanceCountHighReserved : Int4B
84 +0x314 AlpcMessageId : Uint4B
85 +0x318 AlpcMessage : Ptr32 Void
86 +0x318 AlpcReceiveAttributeSet : Uint4B
87 +0x31c AlpcWaitListEntry : _LIST_ENTRY
88 +0x324 ExitStatus : Int4B
89 +0x328 CacheManagerCount : Uint4B
90 +0x32c IoBoostCount : Uint4B
91 +0x330 IoQoSBoostCount : Uint4B
92 +0x334 IoQoSThrottleCount : Uint4B
93 +0x338 KernelStackReference : Uint4B
94 +0x33c BoostList : _LIST_ENTRY
95 +0x344 DeboostList : _LIST_ENTRY
96 +0x34c BoostListLock : Uint4B
97 +0x350 IrpListLock : Uint4B
98 +0x354 ReservedForSynchTracking : Ptr32 Void
99 +0x358 CmCallbackListHead : _SINGLE_LIST_ENTRY
100 +0x35c ActivityId : Ptr32 _GUID
101 +0x360 SeLearningModeListHead : _SINGLE_LIST_ENTRY
102 +0x364 VerifierContext : Ptr32 Void
103 +0x368 AdjustedClientToken : Ptr32 Void
104 +0x36c WorkOnBehalfThread : Ptr32 Void
105 +0x370 PropertySet : _PS_PROPERTY_SET
106 +0x37c PicoContext : Ptr32 Void
107 +0x380 UserFsBase : Uint4B
108 +0x384 UserGsBase : Uint4B
109 +0x388 EnergyValues : Ptr32 _THREAD_ENERGY_VALUES
110 +0x38c SelectedCpuSets : Uint4B
111 +0x38c SelectedCpuSetsIndirect : Ptr32 Uint4B
112 +0x390 Silo : Ptr32 _EJOB
113 +0x394 ThreadName : Ptr32 _UNICODE_STRING
114 +0x398 SparePointer : Ptr32 Void
115 +0x39c LastExpectedRunTime : Uint4B
116 +0x3a0 HeapData : Uint4B
117 +0x3a4 OwnerEntryListHead : _LIST_ENTRY
118 +0x3ac DisownedOwnerEntryListLock : Uint4B
119 +0x3b0 DisownedOwnerEntryListHead : _LIST_ENTRY
120 +0x3b8 LockEntries : [6] _KLOCK_ENTRY
121 +0x4d8 CmDbgInfo : Ptr32 Void
复制代码
Kthread
Ethread的子结构体,里面的成员相对于Ethread更重要。
结构体成员:
复制代码
1 ntdll!_KTHREAD
2 +0x000 Header : _DISPATCHER_HEADER
3 +0x010 SListFaultAddress : Ptr32 Void
4 +0x018 QuantumTarget : Uint8B
5 +0x020 InitialStack : Ptr32 Void
6 +0x024 StackLimit : Ptr32 Void
7 +0x028 StackBase : Ptr32 Void
8 +0x02c ThreadLock : Uint4B
9 +0x030 CycleTime : Uint8B
10 +0x038 HighCycleTime : Uint4B
11 +0x03c ServiceTable : Ptr32 Void
12 +0x040 CurrentRunTime : Uint4B
13 +0x044 ExpectedRunTime : Uint4B
14 +0x048 KernelStack : Ptr32 Void
15 +0x04c StateSaveArea : Ptr32 _XSAVE_FORMAT
16 +0x050 SchedulingGroup : Ptr32 _KSCHEDULING_GROUP
17 +0x054 WaitRegister : _KWAIT_STATUS_REGISTER
18 +0x055 Running : UChar
19 +0x056 Alerted : [2] UChar
20 +0x058 AutoBoostActive : Pos 0, 1 Bit
21 +0x058 ReadyTransition : Pos 1, 1 Bit
22 +0x058 WaitNext : Pos 2, 1 Bit
23 +0x058 SystemAffinityActive : Pos 3, 1 Bit
24 +0x058 Alertable : Pos 4, 1 Bit
25 +0x058 UserStackWalkActive : Pos 5, 1 Bit
26 +0x058 ApcInterruptRequest : Pos 6, 1 Bit
27 +0x058 QuantumEndMigrate : Pos 7, 1 Bit
28 +0x058 UmsDirectedSwitchEnable : Pos 8, 1 Bit
29 +0x058 TimerActive : Pos 9, 1 Bit
30 +0x058 SystemThread : Pos 10, 1 Bit
31 +0x058 ProcessDetachActive : Pos 11, 1 Bit
32 +0x058 CalloutActive : Pos 12, 1 Bit
33 +0x058 ScbReadyQueue : Pos 13, 1 Bit
34 +0x058 ApcQueueable : Pos 14, 1 Bit
35 +0x058 ReservedStackInUse : Pos 15, 1 Bit
36 +0x058 UmsPerformingSyscall : Pos 16, 1 Bit
37 +0x058 TimerSuspended : Pos 17, 1 Bit
38 +0x058 SuspendedWaitMode : Pos 18, 1 Bit
39 +0x058 SuspendSchedulerApcWait : Pos 19, 1 Bit
40 +0x058 CetUserShadowStack : Pos 20, 1 Bit
41 +0x058 BypassProcessFreeze : Pos 21, 1 Bit
42 +0x058 Reserved : Pos 22, 10 Bits
43 +0x058 MiscFlags : Int4B
44 +0x05c ThreadFlagsSpare : Pos 0, 2 Bits
45 +0x05c AutoAlignment : Pos 2, 1 Bit
46 +0x05c DisableBoost : Pos 3, 1 Bit
47 +0x05c AlertedByThreadId : Pos 4, 1 Bit
48 +0x05c QuantumDonation : Pos 5, 1 Bit
49 +0x05c EnableStackSwap : Pos 6, 1 Bit
50 +0x05c GuiThread : Pos 7, 1 Bit
51 +0x05c DisableQuantum : Pos 8, 1 Bit
52 +0x05c ChargeOnlySchedulingGroup : Pos 9, 1 Bit
53 +0x05c DeferPreemption : Pos 10, 1 Bit
54 +0x05c QueueDeferPreemption : Pos 11, 1 Bit
55 +0x05c ForceDeferSchedule : Pos 12, 1 Bit
56 +0x05c SharedReadyQueueAffinity : Pos 13, 1 Bit
57 +0x05c FreezeCount : Pos 14, 1 Bit
58 +0x05c TerminationApcRequest : Pos 15, 1 Bit
59 +0x05c AutoBoostEntriesExhausted : Pos 16, 1 Bit
60 +0x05c KernelStackResident : Pos 17, 1 Bit
61 +0x05c TerminateRequestReason : Pos 18, 2 Bits
62 +0x05c ProcessStackCountDecremented : Pos 20, 1 Bit
63 +0x05c RestrictedGuiThread : Pos 21, 1 Bit
64 +0x05c VpBackingThread : Pos 22, 1 Bit
65 +0x05c ThreadFlagsSpare2 : Pos 23, 1 Bit
66 +0x05c EtwStackTraceApcInserted : Pos 24, 8 Bits
67 +0x05c ThreadFlags : Int4B
68 +0x060 Tag : UChar
69 +0x061 SystemHeteroCpuPolicy : UChar
70 +0x062 UserHeteroCpuPolicy : Pos 0, 7 Bits
71 +0x062 ExplicitSystemHeteroCpuPolicy : Pos 7, 1 Bit
72 +0x063 Spare0 : UChar
73 +0x064 SystemCallNumber : Uint4B
74 +0x068 FirstArgument : Ptr32 Void
75 +0x06c TrapFrame : Ptr32 _KTRAP_FRAME
76 +0x070 ApcState : _KAPC_STATE
77 +0x070 ApcStateFill : [23] UChar
78 +0x087 Priority : Char
79 +0x088 UserIdealProcessor : Uint4B
80 +0x08c ContextSwitches : Uint4B
81 +0x090 State : UChar
82 +0x091 Spare12 : Char
83 +0x092 WaitIrql : UChar
84 +0x093 WaitMode : Char
85 +0x094 WaitStatus : Int4B
86 +0x098 WaitBlockList : Ptr32 _KWAIT_BLOCK
87 +0x09c WaitListEntry : _LIST_ENTRY
88 +0x09c SwapListEntry : _SINGLE_LIST_ENTRY
89 +0x0a4 Queue : Ptr32 _DISPATCHER_HEADER
90 +0x0a8 Teb : Ptr32 Void
91 +0x0b0 RelativeTimerBias : Uint8B
92 +0x0b8 Timer : _KTIMER
93 +0x0e0 WaitBlock : [4] _KWAIT_BLOCK
94 +0x0e0 WaitBlockFill8 : [20] UChar
95 +0x0f4 ThreadCounters : Ptr32 _KTHREAD_COUNTERS
96 +0x0e0 WaitBlockFill9 : [44] UChar
97 +0x10c XStateSave : Ptr32 _XSTATE_SAVE
98 +0x0e0 WaitBlockFill10 : [68] UChar
99 +0x124 Win32Thread : Ptr32 Void
100 +0x0e0 WaitBlockFill11 : [88] UChar
101 +0x138 WaitTime : Uint4B
102 +0x13c KernelApcDisable : Int2B
103 +0x13e SpecialApcDisable : Int2B
104 +0x13c CombinedApcDisable : Uint4B
105 +0x140 QueueListEntry : _LIST_ENTRY
106 +0x148 NextProcessor : Uint4B
107 +0x148 NextProcessorNumber : Pos 0, 31 Bits
108 +0x148 SharedReadyQueue : Pos 31, 1 Bit
109 +0x14c QueuePriority : Int4B
110 +0x150 Process : Ptr32 _KPROCESS
111 +0x154 UserAffinity : _GROUP_AFFINITY
112 +0x154 UserAffinityFill : [6] UChar
113 +0x15a PreviousMode : Char
114 +0x15b BasePriority : Char
115 +0x15c PriorityDecrement : Char
116 +0x15c ForegroundBoost : Pos 0, 4 Bits
117 +0x15c UnusualBoost : Pos 4, 4 Bits
118 +0x15d Preempted : UChar
119 +0x15e AdjustReason : UChar
120 +0x15f AdjustIncrement : Char
121 +0x160 AffinityVersion : Uint4B
122 +0x164 Affinity : _GROUP_AFFINITY
123 +0x164 AffinityFill : [6] UChar
124 +0x16a ApcStateIndex : UChar
125 +0x16b WaitBlockCount : UChar
126 +0x16c IdealProcessor : Uint4B
127 +0x170 ReadyTime : Uint4B
128 +0x174 SavedApcState : _KAPC_STATE
129 +0x174 SavedApcStateFill : [23] UChar
130 +0x18b WaitReason : UChar
131 +0x18c SuspendCount : Char
132 +0x18d Saturation : Char
133 +0x18e SListFaultCount : Uint2B
134 +0x190 SchedulerApc : _KAPC
135 +0x190 SchedulerApcFill0 : [1] UChar
136 +0x191 ResourceIndex : UChar
137 +0x190 SchedulerApcFill1 : [3] UChar
138 +0x193 QuantumReset : UChar
139 +0x190 SchedulerApcFill2 : [4] UChar
140 +0x194 KernelTime : Uint4B
141 +0x190 SchedulerApcFill3 : [36] UChar
142 +0x1b4 WaitPrcb : Ptr32 _KPRCB
143 +0x190 SchedulerApcFill4 : [40] UChar
144 +0x1b8 LegoData : Ptr32 Void
145 +0x190 SchedulerApcFill5 : [47] UChar
146 +0x1bf CallbackNestingLevel : UChar
147 +0x1c0 UserTime : Uint4B
148 +0x1c4 SuspendEvent : _KEVENT
149 +0x1d4 ThreadListEntry : _LIST_ENTRY
150 +0x1dc MutantListHead : _LIST_ENTRY
151 +0x1e4 AbEntrySummary : UChar
152 +0x1e5 AbWaitEntryCount : UChar
153 +0x1e6 AbAllocationRegionCount : UChar
154 +0x1e7 SystemPriority : Char
155 +0x1e8 LockEntries : Ptr32 _KLOCK_ENTRY
156 +0x1ec PropagateBoostsEntry : _SINGLE_LIST_ENTRY
157 +0x1f0 IoSelfBoostsEntry : _SINGLE_LIST_ENTRY
158 +0x1f4 PriorityFloorCounts : [16] UChar
159 +0x204 PriorityFloorCountsReserved : [16] UChar
160 +0x214 PriorityFloorSummary : Uint4B
161 +0x218 AbCompletedIoBoostCount : Int4B
162 +0x21c AbCompletedIoQoSBoostCount : Int4B
163 +0x220 KeReferenceCount : Int2B
164 +0x222 AbOrphanedEntrySummary : UChar
165 +0x223 AbOwnedEntryCount : UChar
166 +0x224 ForegroundLossTime : Uint4B
167 +0x228 GlobalForegroundListEntry : _LIST_ENTRY
168 +0x228 ForegroundDpcStackListEntry : _SINGLE_LIST_ENTRY
169 +0x22c InGlobalForegroundList : Uint4B
170 +0x230 QueuedScb : Ptr32 _KSCB
171 +0x238 NpxState : Uint8B
172 +0x240 ThreadTimerDelay : Uint4B
173 +0x244 ThreadFlags3 : Int4B
174 +0x244 BamQosLevel : Pos 0, 8 Bits
175 +0x244 PpmPolicy : Pos 8, 2 Bits
176 +0x244 ThreadFlags3Reserved2 : Pos 10, 22 Bits
177 +0x248 AbWaitObject : Ptr32 Void
178 +0x24c ReservedPreviousReadyTimeValue : Uint4B
179 +0x250 KernelWaitTime : Uint8B
180 +0x258 UserWaitTime : Uint8B
181 +0x260 Spare29 : [3] Uint4B
182 +0x26c EndPadding : [5] Uint4B
|
-
-
ReadProcess函数
评分
-
参与人数 34 | HB +33 |
THX +13 |
收起
理由
|
禽大师
| + 1 |
|
|
再来壹瓶
| |
+ 1 |
[吾爱汇编论坛52HB.COM]-学破解防破解,知进攻懂防守! |
消逝的过去
| |
+ 1 |
|
花盗睡鼠
| + 2 |
+ 1 |
[吾爱汇编论坛52HB.COM]-学破解防破解,知进攻懂防守! |
DDK4282
| + 1 |
|
[吾爱汇编论坛52HB.COM]-感谢楼主热心分享,小小评分不成敬意! |
霍华德
| + 1 |
+ 1 |
|
一路走来不容易
| + 1 |
|
|
虚心学习
| + 1 |
|
|
帝都骑士
| + 1 |
|
|
爱汇编爱汇编
| + 1 |
|
[吾爱汇编论坛52HB.COM]-软件反汇编逆向分析,软件安全必不可少! |
极速菜
| |
+ 1 |
|
娄胖胖
| + 1 |
|
|
sjtkxy
| + 1 |
|
|
冷亦飞
| + 1 |
|
|
temp
| + 1 |
|
|
fengyuan0128
| + 1 |
|
|
玖霊後
| + 1 |
|
【不符版规】您所发布的内容,不符合版规要求。5HB为系统默认发帖奖励,现已撤销。 |
xgbnapsua
| |
+ 1 |
|
罗密欧
| + 1 |
|
|
272089993
| + 1 |
|
|
逆君
| + 1 |
+ 1 |
[吾爱汇编论坛52HB.COM]-软件反汇编逆向分析,软件安全必不可少! |
让IP飘一会
| + 3 |
+ 1 |
[吾爱汇编论坛52HB.COM]-软件反汇编逆向分析,软件安全必不可少! |
wbxs2077
| + 2 |
+ 1 |
[吾爱汇编论坛52HB.COM]-感谢楼主热心分享,小小评分不成敬意! |
szukodf
| + 1 |
|
[吾爱汇编论坛52HB.COM]-软件反汇编逆向分析,软件安全必不可少! |
king51999
| |
+ 1 |
[吾爱汇编论坛52HB.COM]-学破解防破解,知进攻懂防守! |
liugu0hai
| + 1 |
|
[吾爱汇编论坛52HB.COM]-吃水不忘打井人,给个评分懂感恩! |
ldljlzw
| + 1 |
|
|
河图
| + 1 |
+ 1 |
[吾爱汇编论坛52HB.COM]-软件反汇编逆向分析,软件安全必不可少! |
zxjzzh
| |
+ 1 |
[吾爱汇编论坛52HB.COM]-学破解防破解,知进攻懂防守! |
zwj00544
| + 1 |
+ 1 |
|
成丰羽
| + 1 |
|
[吾爱汇编论坛52HB.COM]-感谢楼主热心分享,小小评分不成敬意! |
bnjzzheng
| + 1 |
|
[吾爱汇编论坛52HB.COM]-吃水不忘打井人,给个评分懂感恩! |
playboy
| + 2 |
|
|
我是好人
| + 1 |
|
[吾爱汇编论坛52HB.COM]-软件反汇编逆向分析,软件安全必不可少! |
查看全部评分
|